Hi.
I'll be using UbuntuMATE, which is a branch of Ubuntu, but this tutorial should apply to any distro or OS, just the steps of downloading and installing the software might be a little different.
Step 1 : Installing MonoDevelop.
First, we'll get our IDE rolling.
-Download MonoDevelop
Just follow the (very nicely) written guide on their website and install MonoDevelop.
OR
On the latest Ubuntu/UbuntuMATE, you only need to do the following in terminal:
Additionally to install git tools, you can do the following after installing it -
Step 2: Downloading SFML.
Now that we have our IDE, let's get our SFML libraries.
-Download the latest SFML package.
Make sure you get the correct package for your OS and your architecture. Read the notes on the website for more info. I suggest going 32-bit packages if you're using Windows.
Alright! We have downloaded our file.
We'll begin by unzipping it.
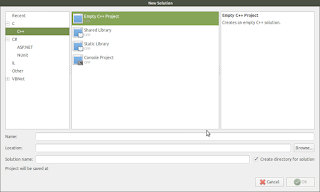
Click C on the left, and select C++ and click on Empty C++ Project.
(I'll be using C++, you can use C# or other bindings)
Enter the Name of the project and the location on the disk.
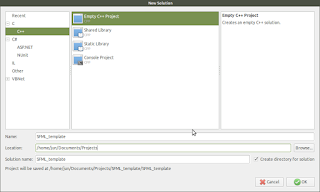
Hit OK.
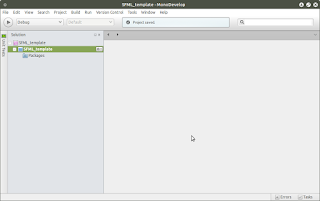
If it's completely blank, just do View > Visual Design
Add a new file in our solution, make sure it's a C++ file. Call it main.cpp
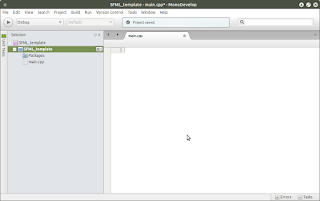
Copypaste the following code from SFML Linux Guide:
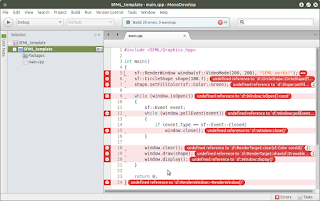
Oh noes! MonoDevelop doesn't know where the SFML files are, and thus cannot comprehend the code and throws out a bunch of errors. Let's fix it.
Go to Project > SFML_template options (Or yoursolutionname options)
On the left side, click Code Generation under Build
Click the Paths tab.
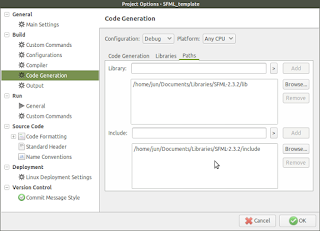
Now, add the path to your
SFML-x.x.x/lib under Library
and
SFML-x.x.x/include under Include
Click the Libraries tab.
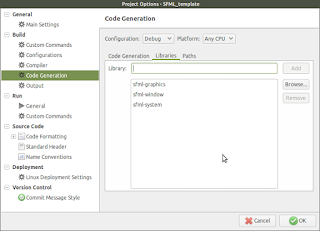
type in one at a time these three libraries and add them.
Viola!
Hit OK and Save your project.
And run it!
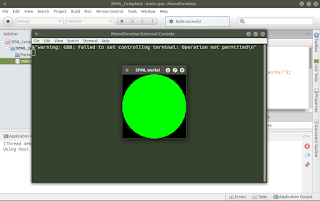
Yay! We've successfully set up MonoDevelop C++ with SFML. Enjoy, and code away~
PS: If you're on linux and mono throws an error regarding gdb on building, just
sudo apt-get install gdb and you should be good.
Same goes with gcc/g++ if you're getting errors on compiling.
Any questions/comments? Want to thank me or insult me? Want to have a casual chat? Go on, comment below!
Have a good day.
~Jun
I'll be using UbuntuMATE, which is a branch of Ubuntu, but this tutorial should apply to any distro or OS, just the steps of downloading and installing the software might be a little different.
Step 1 : Installing MonoDevelop.
First, we'll get our IDE rolling.
-Download MonoDevelop
Just follow the (very nicely) written guide on their website and install MonoDevelop.
OR
On the latest Ubuntu/UbuntuMATE, you only need to do the following in terminal:
sudo apt-get update
sudo apt-get upgrade
sudo apt-get install mono-complete
sudo apt-get install monodevelop-nunitAnd that's that! It should take a few moments and you should have the MonoDevelop IDE installed.
Additionally to install git tools, you can do the following after installing it -
sudo apt-get install monodevelop-versioncontrolLet's test it by running it via terminal. Type in teriminal:
monodevelopViola! If you see the IDE pop up, you're golden. Let's move on to the next step.
Step 2: Downloading SFML.
Now that we have our IDE, let's get our SFML libraries.
-Download the latest SFML package.
Make sure you get the correct package for your OS and your architecture. Read the notes on the website for more info. I suggest going 32-bit packages if you're using Windows.
Alright! We have downloaded our file.
We'll begin by unzipping it.
If you're on linux, chances are you already have an archive manager. Otherwise, I suggest getting 7zip.
The SFML folder should have at least two folders, namely include and lib.
Now, move the SFML folder to somewhere you will remember.
I suggest /home/<yourusername>/Libraries/ or C:/SFML
And we're done with step 2!
Step 3: Setting up MonoDevelop.
Launch MonoDevelop.
We'll now create a new Solution.
File > New > Solution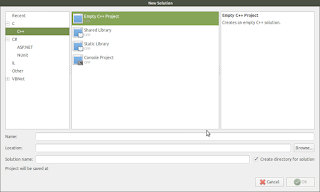
Click C on the left, and select C++ and click on Empty C++ Project.
(I'll be using C++, you can use C# or other bindings)
Enter the Name of the project and the location on the disk.
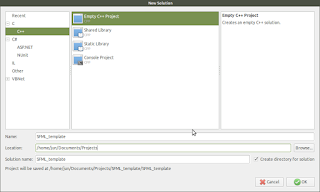
Hit OK.
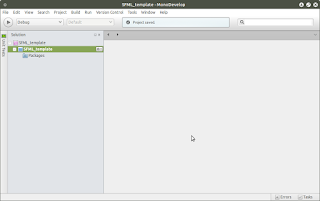
If it's completely blank, just do View > Visual Design
Add a new file in our solution, make sure it's a C++ file. Call it main.cpp
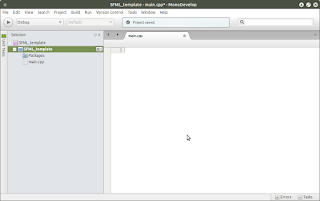
Copypaste the following code from SFML Linux Guide:
#include <SFML/Graphics.hpp>Try to build it.
int main()
{
sf::RenderWindow window(sf::VideoMode(200, 200), "SFML works!");
sf::CircleShape shape(100.f);
shape.setFillColor(sf::Color::Green);
while (window.isOpen())
{
sf::Event event;
while (window.pollEvent(event))
{
if (event.type == sf::Event::Closed)
window.close();
}
window.clear();
window.draw(shape);
window.display();
}
return 0;
}
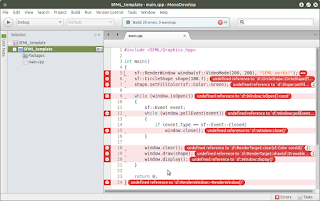
Oh noes! MonoDevelop doesn't know where the SFML files are, and thus cannot comprehend the code and throws out a bunch of errors. Let's fix it.
Go to Project > SFML_template options (Or yoursolutionname options)
On the left side, click Code Generation under Build
Click the Paths tab.
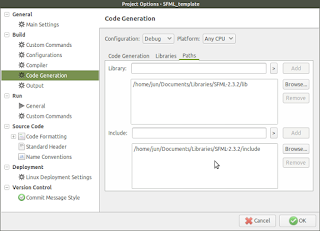
Now, add the path to your
SFML-x.x.x/lib under Library
and
SFML-x.x.x/include under Include
Click the Libraries tab.
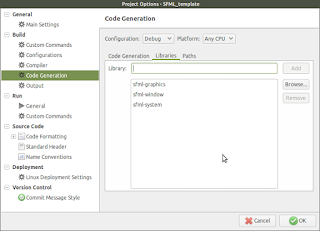
type in one at a time these three libraries and add them.
sfml-graphics
sfml-window
sfml-systemIf you need any other libraries in your project, just add them here.
Viola!
Hit OK and Save your project.
And run it!
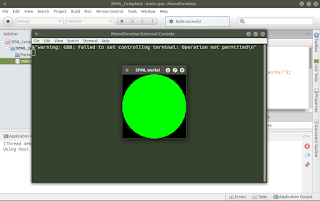
Yay! We've successfully set up MonoDevelop C++ with SFML. Enjoy, and code away~
PS: If you're on linux and mono throws an error regarding gdb on building, just
sudo apt-get install gdb and you should be good.
Same goes with gcc/g++ if you're getting errors on compiling.
Any questions/comments? Want to thank me or insult me? Want to have a casual chat? Go on, comment below!
Have a good day.
~Jun
No comments:
Post a Comment